Become a Python Developer.
Starting with the basics, ending at mastery. We've got you covered every step of the way.
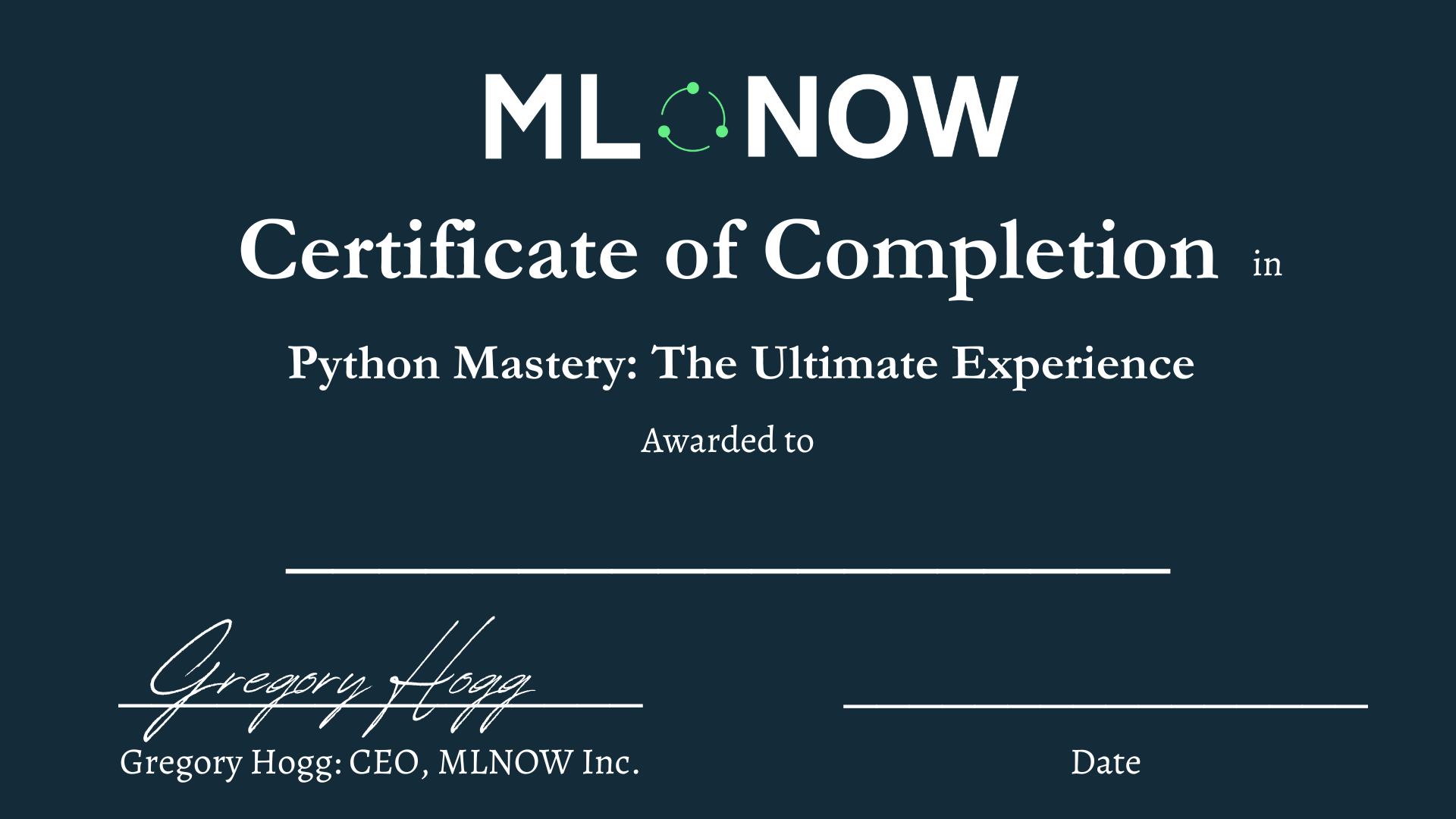
Get a Certificate That Means Something.

The Best Learning Environment.
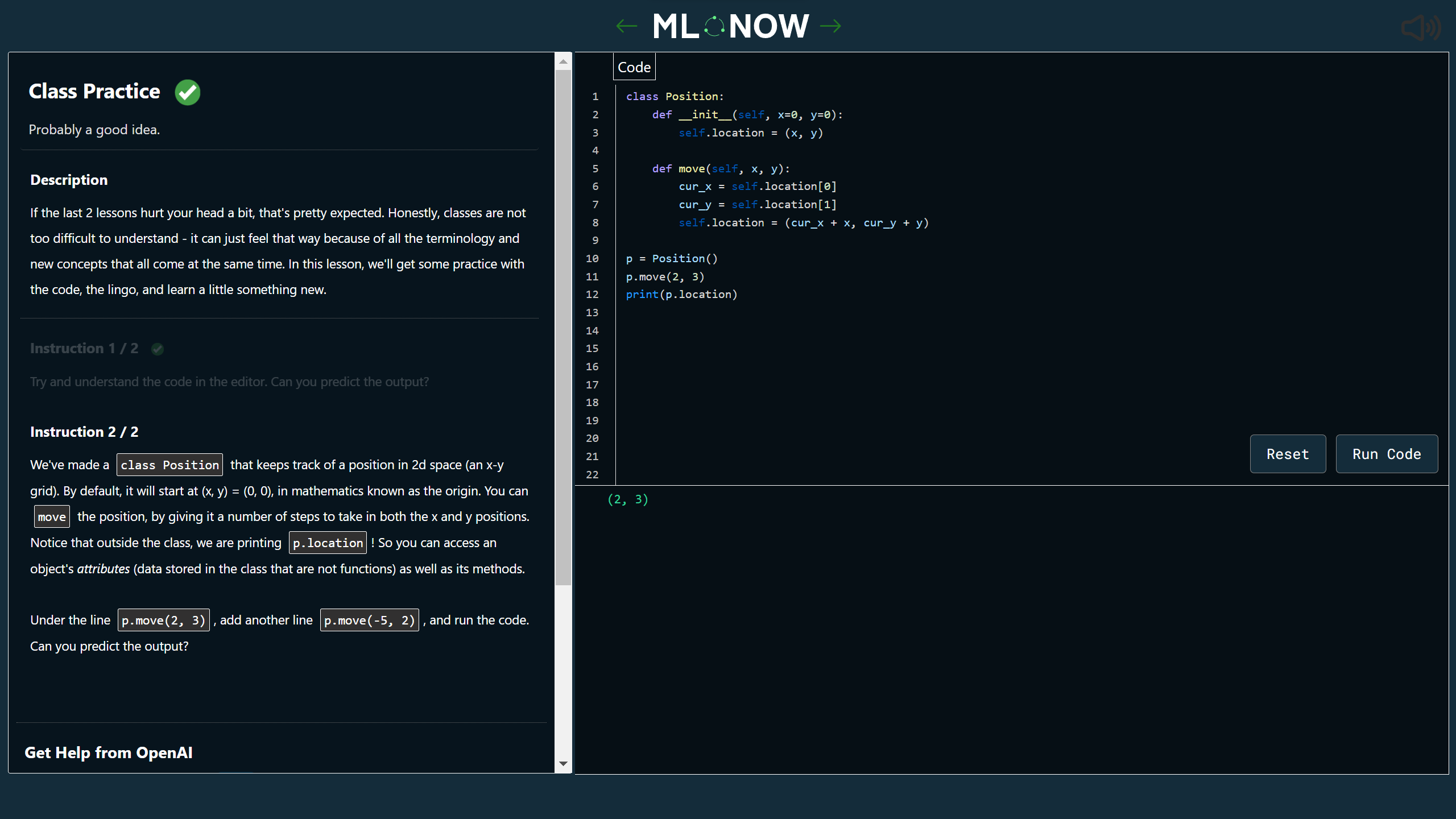
And finally, our Course Material.
-
Chapter 1: Exploring the Environment
Get introduced to Python and learn fundamental programming concepts such as Variables, Data Types, and Mathematical Operations.
💻1: Introduction to Python ... Gotta start somewhere.
💻2: Python Syntax ... The rules of Python.
🧠3: Recall 1
💻4: Python Variables ... They can vary. But they don't have to.
💻5: Python Data Type: String ... best_platform = 'ml_now'
💻6: Python Data Type: Integer ... Numbers like -12, 0, and 4.
💻7: Integer Operations ... 1 + 1 == 2. Last we checked anyway...
💻8: Python Data Type: Float ... 1.0 + 1.0 == 2.0
🧠9: Recall 2
💻10: Integer Division ... Fun fact: 7 // 3 == 2... huh?
💻11: Modulus Operation ... 5 % 2 == 1 (the remainder)
🧠12: Recall 3
💻13: BEDMAS ... (5 + 3) * 2 == 16
💻14: Python Data Type: Boolean ... True & False
🧠15: Recall 4
💻16: Special Characters ... In Python, text is stored in strings.
💻17: The Type Function ... What's the type???
✏️18: Final Assessment
Chapter 2: Essential Utilities
Learn crucial Python techniques and computer science paradigms such as String Formatting, Conditional Statements, and Logical Programming.
💻1: Variable Names ... There's naming rules. And best practices.
💻2: Comments ... # This is a Python comment
🧠3: Recall 1
💻4: F-Strings ... var = 'awesome'... f'ML NOW is {var}'
💻5: String Format ... 'Greg is {0}'.format('Really cool')
🧠6: Recall 2
💻7: Updating Numerical Variables ... There's cool shortform notation.
💻8: Type Conversion ... int(5.75) == 5
💻9: len( ) ... Size matters.
💻10: Conditional Statements ... 3 < 5 == True
🧠11: Recall 3
💻12: And & Or ... (True or False) == True
✏️13: Final Assessment
Chapter 3: Conditional Flow
Master Conditional Execution and If Statements in Python: Learn If, If-Else, and If-Elif-Else evaluation chains.
Chapter 4: Loops
Take your programming to the next level with Loops and Iteration using For-Loops, While-Loops, and Iterables such as Lists.
💻1: We Need Loops ... We really do...
💻2: For Loops Part 1 ... Do it again and again.
💻3: For Loops Part 2 (Range) ... There's an off by one which is annoying but useful.
🧠4: Recall 1
💻5: Lists ... The most common Python data structure.
💻6: Lists and Loops ... Loop through those lists.
💻7: List Indices ... They start at 0. Annoying, but it's consistent.
🧠8: Recall 2
💻9: While Loops ... while on_the_site: consider_upgrading = True
🧠10: Recall 3
💻11: Breaking out of loops ... Get out now!!!
✏️12: Final Assessment
Chapter 5: Data Structures
Become an expert in Python's fundamental Data Structures - Lists, Dictionaries, Sets, and Tuples.
💻1: Lists Part 1 ... Haven't we already seen these?
💻2: Lists: Part 2 ... They're really useful so we're gonna teach a lot about them.
💻3: Lists: Part 3 (Slicing) ... Not as good as pizza, but often more useful.
💻4: Lists: Part 4 (Split) ... One of our favorite functions.
🧠5: Recall 1
💻6: Dictionaries: Part 1 ... They're basically in-memory JSON files.
💻7: Dictionaries: Part 2 (Looping) ... We can loop through a dictionary!
🧠8: Recall 2
💻9: Just "in" ... It was a joke about the news... Just In? Get it?
💻10: Tuples ... Less useful lists... but they'll come up every now and then.
💻11: Sets ... Honestly, you might never see these again. But you might.
✏️12: Final Assessment
Chapter 6: Functions
Truly master Python Functions - the ultimate way to write code that you can use time and time again.
💻1: Introduction to Functions ... Be patient with these things, they can be tricky.
💻2: User-Defined Functions ... We'll make our own!
💻3: Function Practice ... We definitely need some.
💻4: More Function Practice ... Trust, it's worth it.
🧠5: Recall 1
💻6: Return and None ... Some functions return things. Others don't.
💻7: Side Effects ... Functions can affect the outside.
💻8: Return vs Side Effect ... You can often do either. Or both.
🧠9: Recall 2
💻10: Default Values ... Default values for Parameters.
💻11: Keyword Arguments ... A useful tool when calling functions.
🧠12: Recall 3
💻13: Setting a Variable to Another ... Python shares memory when it can.
💻14: Variable Scope ... Ah it can be pretty tricky, take your time!
💻15: Variable Scope Practice ... Honestly, this lesson is a lot more than just practice.
🧠16: Recall 4
✏️17: Final Assessment
Chapter 7: Classes
Gain a complete understanding of Python Classes, Objects, and the essential Object-Oriented Programming Paradigm.
💻1: Introduction to Classes ... Our own custom data structures. We make a Robot.
💻2: Classes Continued ... This lesson is basically just a ton of lingo. But we need it.
💻3: Class Practice ... Probably a good idea.
🧠4: Recall 1
💻5: Built-in Data Structures are Classes ... Cool story bro..?
💻6: Method Overriding ... They're actually really cool.
✏️7: Final Assessment
Chapter 8: File Management
Become a File Processing Specialist in Python by Reading, Writing, and Appending to Text, JSON, and CSV files. Plus, learn how to import Python Libraries and Modules to re-use code blocks.
💻1: File Input / Output 1 ... IO
💻2: File Input / Output 2 ... Most courses skip in-depth file management. You're welcome.
💻3: File Input / Output 3 ... Not sure what to write here. So... hey there.
🧠4: Recall 1
💻5: JSON Files 1 ... Basically just a file storing a dictionary.
💻6: JSON Files 2 ... We'll read them into a dictionary.
💻7: CSV Files 1 ... Comma Separated Values.
💻8: CSV Files 2 ... Comma Separated Values. Wait we already wrote that above.
💻9: Python Files ... Import!
🧠10: Recall 2
✏️11: Final Assessment
Chapter 9: Bonus!
Unlock your inner Python God by mastering Exception Handling, List Comprehension, Subclassing, and Python's most advanced tools.
💻1: Try-Except ... Gotta be ready for potential errors.
💻2: Try-Except 2 ... We'll learn how not to have any big errors.
💻3: Try-Except 3 ... Here we'll target specific errors.
🧠4: Recall 1
💻5: List Comprehension ... Super-fast list making.
💻6: Zip ... Iterating over multiple things at once.
💻7: Dictionary Comprehension ... Super-fast dictionary making
🧠8: Recall 2
💻9: Enumerate ... Looping over indices and items.
💻10: Subclassing ... Classes can have parents and children. It's complicated.
💻11: Copy ... Copy that!
🧠12: Recall 3
💻13: Higher Level Functions 1 ... Passing a function to a function.
💻14: Higher Level Functions 2 ... Returning a function from a function.
💻15: Lambda Functions ... Functions without names. So... anonymous functions.
🧠16: Recall 4
✏️17: Final Assessment